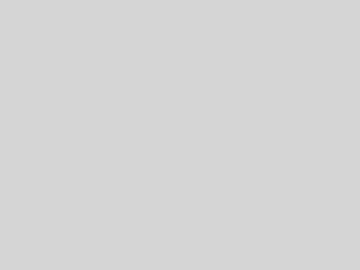
Java 8 – Optional class in detail
In this article, we will discuss Optional class in detail and understand why it is needed/introduced in Java 1.8 version and how to avoid/handle (or Read More
In this article, we will discuss Optional class in detail and understand why it is needed/introduced in Java 1.8 version and how to avoid/handle (or Read More
In this article, we will see how to reverse a String in place using traditional & Java 8 Stream approaches 1. Traditional approach : ReverseStringInPlace.java Read More
In this article, we will discuss how to find and print common & uncommon elements from 2 Lists (or ArrayList) There are different ways to Read More
In this article, we will discuss how to sort a HashMap by its Values first and then by its Keys 1. Sorting HashMap by Value Read More
In this article, we will discuss how to write Java code to create Thread-safe Singleton class in a Multi-threaded environment Before starting to write code Read More
In this article, we will discuss how to find the desired result from the Integer[] arrays for the problem statement given below 1. Problem statement Read More
In this article, we will discuss how to find employee count in every department using Java 8 Stream API Find employee count in each department Read More
In this article, we will discuss how to check if a String is an Integer or not using different String literals Check String is an Read More
In this article, we will discuss 2 important methods of Thread class – start() & run() 1. Thread methods : There are several methods in Read More
In this article, we will discuss important methods and constructors of a Thread class 1. Thread : 1.1 Creating a Thread : There are 2 Read More
In this article, we will discuss difference between extending a Thread class and implementing a Runnable interface for creating a new Thread 1. Thread class Read More
In this article, we will discuss different ways to create or spawn a Thread in Java 1. Creating a Thread : There are 2 ways Read More
In this article, we will discuss the concepts of Thread in Java 1. Task and Multi-tasking : Before understanding Thread, first we will try to Read More
In this article, we will discuss how to find and count duplicate values in a Map or HashMap Find & Count duplicate values in a Read More
In this article, we will discuss how to reverse a Queue in different ways Reverse a Queue : There are different ways to reverse a Read More
In this article, we will discuss how to find maximum & minimum Map Key & Value 1. Maximum & Minimum Map Key : FindMaximumMinimumMapKey.java Output Read More
In this article, we will discuss how to remove an entry based on the Value in a HashMap using Java 8 Stream Remove an entry Read More
In this article, we will discuss how to find an entry based on the Value in a HashMap using Java 8 Stream Find an entry Read More
In this article, we will discuss how to remove an entry based on the Key in a HashMap using Java 8 Stream Remove an entry Read More
In this article, we will discuss how to find an entry based on the Key in a HashMap using Java 8 Stream Find an entry Read More